Create a Website with HTML5
Lesson #2
The Basic HTML Page
All HTML web pages begin with a simple structure shown below:
<!DOCTYPE html>
<html>
<head>
</head>
<body>
</body>
</html>
The top line of any web page (shown in red) will be occupied by your doctype, which simply tells the viewing appliance what kind of media to expect.
Next, note that there are 2 sections to the structure nested within an open and close HTML tag.(shown in blue)
<html>2 page sections</html>
The first section enclosed in these 2 tags
<head>Head Section</head>
is called the Head section and will contain information for the visiting browser. (shown in green)
The second section enclosed in these 2 tags
<body>Body section</body>
is called the Body section and will contain all the code for the text and pictures that will appear on your web page.
Code without DOCTYPE:
<html>
<head>
</head>
<body>
</body>
</html>
Exercise #1
In Exercise #1 we'll create our first HTML page, mytemplate.html. We'll use this as a template to create the 3 page website.
Copy and paste the code shown below into the top text editor window on the right.
<!DOCTYPE html>
<html>
<head>
</head>
<body>
</body>
</html>
Save the HTML code in the top text editor, in the C:/htdocs folder, as mytemplate.html
Note: The doctype tag is case sensitive, so always type it the way it is shown here. Nothing else in html is case sensitive.
Exercise #2
In this exercise we'll paste some code into the bottom text editor and save it to create our style sheet.
Copy and paste the code shown below into the bottom text editor window on the right.
* {
margin: 0 0 0 0;
padding: 0 0 0 0
}
body {
max-width: 1200px;
margin: 0 auto;
color: #0e0e0e;
background: #AAD5FF;
font-family: Arial, verdana, tahoma , serif;
font-size: 18px;
font-style: normal;
font-weight: 400
}
Save the CSS code in the bottom text editor, in the C:/htdocs folder as mystyle.css.
max-width: 1200px;
Even though we're building liquid web pages, we do not want our design to spread out to 1600 or 1900 pixels wide on high resolution machines. (This is, of course a matter of taste. )
You can control the maximum width you want your page to display at using this simple line of code.
If you're working on a machine with a screen resolution of over 1900 pixels you can experiment by changing the 1200px to a higher number. Determine how much you want your design to spread out.
The line below max-width, margin: 0 auto; centers the display in the browser window.
If you were to look at your new web page with a browser at this point, you would see a blank white screen.
That will change once the HTML page can access the style sheet.
A background color was added in the style sheet using this code background: #AAD5FF;
We also set our default font properties under the body selector to keep from adding them every time we add a new text element.
Exercise #3
In this exercise we'll add some information to the head section of the HTML page including a line that links the web page to the style sheet.
Copy and paste the code shown below into the top text editor window on the right. Place it below the <head> tag and above the </head> tag.
<title>Title of page goes here</title>
<meta name="author" content="your name here">
<meta charset="utf-8">
<meta name="viewport" content="user-scalable=no, width=device-width">
<meta name="DESCRIPTION" content="Place description of page here">
<meta name="KEYWORDS" content="Place keywords or phrases here">
<link rel="stylesheet" href="mystyle.css" type="text/css">
HELP: To create space under a tag, place your cursor at the end of the closing bracket and press Enter a couple of times. In this case you would place your cursor as shown here. <head>|
Save mytemplate.html
We add 7 lines of code <title></title>, meta charset, viewport, author, description and meta keywords that instruct the browser and help search engines to gather information about your site for indexing.
The last line of code in the block links the web page to the style sheet.
Exercise #4
In this exercise we'll add something to the web page that you can see.
Copy and paste the code shown below into the top text editor window on the right. Place it below the <body> tag and above the </body> tag.
<div class="outline">
</div>
Save mytemplate.html
Copy and paste the code shown below into the bottom text editor window on the right below the last entry.
div.outline {
width: 96%;
float: left;
padding: 1% 2%;
margin: 1% 0;
border-radius: 10px;
background: #E6ECFF;
box-shadow: 5px 5px 10px #202020;
height: 400px
}
Save mystyle.css
Now we can preview the web page in a browser.
Read Help Before You Preview!!
HELP: Some with very basic computer knowledge will struggle with navigating the browser at first.
You can return to the lesson after previewing by clicking the Back arrow, top left of browser.
One click takes you to the Folder Index.
The second click takes you to the lesson.
Preview the web page.
HELP: To Preview your web page type: file:///c:/htdocs/ in the URL box at the top and hit enter. If you've followed directions to this point you will see your web page mytemplate.html in the Folder Index that pops up. Just click on the web page file to open it in the browser..
You should see something like this:
Note: You may need to widen the browser window to see the shadow on the right side of the outline division.
Also, the height setting is temporary. We'll remove after we start adding elements inside the outline division.
Adding Attributes to a Tag
When adding attributes to any html tag they are placed as shown:
<p attributes are placed here></p>
Examples:
<div class="outline"></div>
<p style="color: red"></p>
<meta name="description">
<link rel="stylesheet">
Focus at this point on learning to recognize the open and close tag for each element that is added to your web page. The closing tag always includes a forward slash. </html></head></body>
Almost all HTML tags come in pairs. There are some exceptions.
You should be able to find 3 exceptions in the code placed so far.
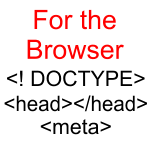
In Lesson #3 we'll add a heading to the page.
If you think you're ready Proceed to Lesson #3